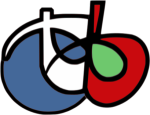 |
OTB
9.1.1
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbSpot5SensorModel_h
22 #define otbSpot5SensorModel_h
32 #include <itkMatrix.h>
33 #include "itkVector.h"
34 #include <itkVersor.h>
83 double heightAboveEllipsoid)
const;
127 const std::vector<Point3DType>& V,
128 const std::vector<double>& T)
const;
139 const std::vector<Point3DType>& V,
140 const std::vector<double>& T)
const;
207 itk::Point<double, 3>
EcefToWorld(
const itk::Point<double, 3> & ecefPoint)
const;
216 itk::Point<double, 3>
WorldToEcef(
const itk::Point<double, 3> & worldPoint)
const;
243 virtual bool insideImage(
const itk::Point<double, 2> point,
double epsilon)
const
245 return (point[0] >= -epsilon) &&
247 (point[1] >= -epsilon) &&
255 static constexpr
double C = 299792458;
itk::Vector< double, 3 > Vector3DType
itk::Point< double, 2 > Point2DType
SPOT5 sensor class, based on OSSIM Spot5SensorModel with ITK/GDAL implementation.
otb::GeocentricTransform< otb::TransformDirection::INVERSE, double >::Pointer m_EcefToWorldTransform
itk::Matrix< double, 3, 3 > MatrixType
itk::SmartPointer< Self > Pointer
Point3DType GetVelocityEcf(double time) const
Get the 3D velocity of the sensor at time (interpolation of velocity samples vector from metadata).
ContinuousIndexType Point2DToIndex(const itk::Point< double, 2 > point) const
Convert 2Dpoint to 2DContinuousIndex.
Point3DType GetAttitude(double time) const
Get the Attitude of the sensor at time (interpolation of attitude samples vector from metadata).
void InitBilinearTransform()
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
otb::GeocentricTransform< otb::TransformDirection::FORWARD, double >::Pointer m_WorldToEcefTransform
BilinearProjection::Pointer m_BilinearProj
PolygonType::Pointer m_ImageRect
virtual ~Spot5SensorModel()=default
virtual bool insideImage(const itk::Point< double, 2 > point, double epsilon) const
Check if point is inside image.
void GetPixelLookAngleXY(unsigned int line, double &psiX, double &psiY) const
Get look angles on X and Y axis of the sensor at line (interpolation of angles samples vector from me...
This structure is used to handle Ephemeris information.
Point3DType GetBilinearInterpolation(const double &time, const std::vector< Point3DType > &V, const std::vector< double > &T) const
Compute the bilinear interpolation from a 3D point vector with a double time vector.
Point3DType LineSampleToWorld(Point2DType imPt) const
Transform image point (col, row) to world point (lat,lon,hgt).
Spot5SensorModel(const std::string &productType, const Spot5Param &Spot5Param)
Superclass::ContinuousIndexType ContinuousIndexType
PolygonType::ContinuousIndexType ContinuousIndexType
itk::Point< double, 3 > WorldToEcef(const itk::Point< double, 3 > &worldPoint) const
Coordinate transformation from geographic to ECEF.
itk::Point< double, 3 > EcefToWorld(const itk::Point< double, 3 > &ecefPoint) const
Coordinate transformation from ECEF to geographic.
Spot5SensorModel & operator=(const Spot5SensorModel &)=delete
Point2DType WorldToLineSample(const Point3DType &inGeoPoint) const
Transform world point (lat,lon,hgt) to image point (col,row).
PolygonType::Pointer m_GroundRect
itk::SmartPointer< Self > Pointer
static constexpr double C
Point3DType NearestIntersection(const Ephemeris &imRay, double offset) const
Compute the nearest intersection (world point) between the ray and the ellipsoid.
Point3DType LineSampleHeightToWorld(Point2DType imPt, double heightAboveEllipsoid) const
Transform image point (col, row) with a height to world point (lat,lon,hgt).
void UpdateImageMetadata(ImageMetadata &imd)
Update a ImageMetadata object with the stored Spot5Param and GCPs, possibly modified from the origina...
MatrixType ComputeSatToOrbRotation(double t) const
Compute SatToOrb matrix with an input time.
Point3DType GetLagrangeInterpolation(const double &time, const std::vector< Point3DType > &V, const std::vector< double > &T) const
Compute the lagrange interpolation from a 3D point vector with a double time vector.
Point3DType GetPositionEcf(double time) const
Get the 3D position of the sensor at time (interpolation of position samples vector from metadata).
This class represent a 2D polygon.
std::string m_ProductType
Point3DType IntersectRay(const Ephemeris &imRay) const
Compute world point intersected by image ray.
Spot5 sensors parameters.
ContinuousIndexType Point3DToIndex(const itk::Point< double, 3 > point) const
Convert 3Dpoint to 2DContinuousIndex.
Ephemeris ImagingRay(Point2DType imPt) const
Compute a ray from sensor position and image point.
itk::Point< double, 3 > Point3DType