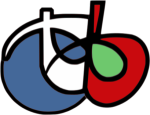 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbImageMetadata_h
22 #define otbImageMetadata_h
27 #include "OTBMetadataExport.h"
30 #include <boost/any.hpp>
34 #include <unordered_map>
53 template <
class TKey,
class TVal>
56 using Keywordlist = std::unordered_map<std::string, std::string>;
106 const boost::any & operator[](
MDGeom key)
const;
110 const SARParam & GetSARParam()
const;
112 std::string GetProjectedGeometry()
const;
114 std::string GetProjectionWKT()
const;
116 std::string GetProjectionProj()
const;
119 void Add(
MDGeom key,
const boost::any &value);
122 size_t Remove(
MDGeom key);
124 size_t RemoveSensorGeometry();
126 size_t RemoveProjectedGeometry();
129 bool Has(
MDGeom key)
const;
131 bool HasSensorGeometry()
const;
133 bool HasProjectedGeometry()
const;
138 const double & operator[](
MDNum key)
const;
141 void Add(
MDNum key,
const double &value);
144 size_t Remove(
MDNum key);
147 bool Has(
MDNum key)
const;
150 std::string GetKeyListNum()
const;
155 const std::string & operator[](
MDStr key)
const;
158 void Add(
MDStr key,
const std::string &value);
161 size_t Remove(
MDStr key);
164 bool Has(
MDStr key)
const;
167 std::string GetKeyListStr()
const;
178 size_t Remove(
MDL1D key);
181 bool Has(
MDL1D key)
const;
184 std::string GetKeyListL1D()
const;
195 size_t Remove(
MDL2D key);
198 bool Has(
MDL2D key)
const;
212 size_t Remove(
MDTime key);
215 bool Has(
MDTime key)
const;
218 std::string GetKeyListTime()
const;
223 const std::string & operator[](
const std::string & key)
const;
226 void Add(
const std::string& key,
const std::string &value);
229 size_t Remove(
const std::string& key);
232 bool Has(
const std::string& key)
const;
240 std::string ToJSON(
bool multiline=
false)
const;
256 std::vector<unsigned int> GetDefaultDisplay()
const;
331 itk::VariableLengthVector<double> GetAsVector(
MDNum key)
const;
334 bool HasBandMetadata(
MDNum key)
const;
337 bool HasBandMetadata(
MDL1D key)
const;
341 std::vector<std::string> GetBandNames()
const;
345 std::vector<std::string> GetEnhancedBandNames()
const;
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
bool HasSameSensorModel(const ImageMetadataBase &a, const ImageMetadataBase &b)
bool HasSameRPCModel(const ImageMetadataBase &a, const ImageMetadataBase &b)
OTBMetadata_EXPORT bool HasSARSensorMetadata(const ImageMetadata &imd)
OTBCommon_EXPORT std::ostream & operator<<(std::ostream &os, const otb::StringToHTML &str)
Coefficients for RPC model (quite similar to GDALRPCInfo)
OTBMetadata_EXPORT bool HasOpticalSensorMetadata(const ImageMetadata &imd)
This structure handles the list of the GCP parameters.
OTBMetadata_EXPORT void WriteImageMetadataToGeomFile(const ImageMetadata &imd, const std::string &filename)
bool HasSameSARModel(const ImageMetadataBase &a, const ImageMetadataBase &b)