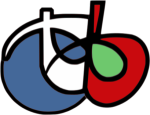 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbSVMMachineLearningModel_h
22 #define otbSVMMachineLearningModel_h
25 #include "itkLightObject.h"
26 #include "itkFixedArray.h"
41 template <
class TInputValue,
class TTargetValue>
66 void Train()
override;
69 void Save(
const std::string& filename,
const std::string& name =
"")
override;
72 void Load(
const std::string& filename,
const std::string& name =
"")
override;
78 bool CanReadFile(
const std::string&)
override;
81 bool CanWriteFile(
const std::string&)
override;
85 itkGetMacro(SVMType,
int);
86 itkSetMacro(SVMType,
int);
88 itkGetMacro(KernelType,
int);
89 itkSetMacro(KernelType,
int);
92 itkGetMacro(TermCriteriaType,
int);
93 itkSetMacro(TermCriteriaType,
int);
95 itkGetMacro(MaxIter,
int);
96 itkSetMacro(MaxIter,
int);
98 itkGetMacro(Epsilon,
double);
99 itkSetMacro(Epsilon,
double);
102 itkGetMacro(Degree,
double);
103 itkSetMacro(Degree,
double);
104 itkGetMacro(OutputDegree,
double);
107 itkGetMacro(Gamma,
double);
108 itkSetMacro(Gamma,
double);
109 itkGetMacro(OutputGamma,
double);
112 itkGetMacro(Coef0,
double);
113 itkSetMacro(Coef0,
double);
114 itkGetMacro(OutputCoef0,
double);
117 itkGetMacro(C,
double);
118 itkSetMacro(C,
double);
119 itkGetMacro(OutputC,
double);
122 itkGetMacro(Nu,
double);
123 itkSetMacro(Nu,
double);
124 itkGetMacro(OutputNu,
double);
127 itkGetMacro(P,
double);
128 itkSetMacro(P,
double);
129 itkGetMacro(OutputP,
double);
131 itkGetMacro(ParameterOptimization,
bool);
132 itkSetMacro(ParameterOptimization,
bool);
145 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
149 void operator=(
const Self&) =
delete;
173 #ifndef OTB_MANUAL_INSTANTIATION
Superclass::InputValueType InputValueType
itk::Statistics::ListSample< TargetSampleType > TargetListSampleType
Superclass::ProbaSampleType ProbaSampleType
Superclass::InputListSampleType InputListSampleType
itk::Statistics::ListSample< InputSampleType > InputListSampleType
MLMSampleTraits< TInputValue >::ValueType InputValueType
cv::Ptr< cv::ml::SVM > m_SVMModel
bool m_ParameterOptimization
Superclass::TargetSampleType TargetSampleType
itk::SmartPointer< const Self > ConstPointer
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
MachineLearningModel< TInputValue, TTargetValue > Superclass
itk::SmartPointer< Self > Pointer
Superclass::InputSampleType InputSampleType
Superclass::TargetListSampleType TargetListSampleType
Superclass::ConfidenceValueType ConfidenceValueType
MachineLearningModel is the base class for all classifier objects (SVM, KNN, Random Forests,...
SVMMachineLearningModel Self
MLMTargetTraits< TTargetValue >::ValueType TargetValueType
OpenCV implementation of SVM algorithm.
MLMTargetTraits< TTargetValue >::SampleType TargetSampleType
Superclass::TargetValueType TargetValueType