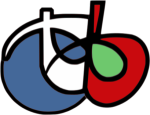 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
22 #ifndef otbLmvmPanSharpeningFusionImageFilter_h
23 #define otbLmvmPanSharpeningFusionImageFilter_h
28 #include "itkNoiseImageFilter.h"
31 #include "itkProgressAccumulator.h"
56 template <
class TPanImageType,
class TXsImageType,
class TOutputImageType,
class TInternalPrecision =
float>
62 typedef itk::ImageToImageFilter<TXsImageType, TOutputImageType>
Superclass;
77 typedef typename itk::Array<TInternalPrecision>
ArrayType;
98 virtual void SetPanInput(
const TPanImageType* image);
99 const TPanImageType* GetPanInput(
void)
const;
101 virtual void SetXsInput(
const TXsImageType* path);
102 const TXsImageType* GetXsInput(
void)
const;
112 void GenerateData()
override;
115 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
119 void operator=(
const Self&);
132 const TInternalPrecision& smoothPanchroPixel,
const typename TPanImageType::PixelType& sharpPanchroPixel)
const
135 for (
unsigned int i = 0; i < stdXsPixel.Size(); ++i)
137 output[i] =
static_cast<typename TOutputImageType::InternalPixelType
>(((sharpPanchroPixel - smoothPanchroPixel) * stdXsPixel[i]));
140 constexpr
size_t OutputSize(
const std::array<size_t, 3> inputsNbBands)
const
142 return inputsNbBands[0];
150 void operator()(
typename TOutputImageType::PixelType& output,
const typename TOutputImageType::PixelType& functor1Pixel,
153 TInternalPrecision scale = 1.;
155 if (std::abs(stdPanchroPixel) > 1e-10)
157 scale = 1.0 / stdPanchroPixel;
161 for (
unsigned int i = 0; i < smoothXsPixel.Size(); ++i)
163 output[i] =
static_cast<typename TOutputImageType::InternalPixelType
>(((functor1Pixel[i] * scale) + smoothXsPixel[i]));
167 constexpr
size_t OutputSize(
const std::array<size_t, 3> inputsNbBands)
const
169 return inputsNbBands[0];
239 #ifndef OTB_MANUAL_INSTANTIATION
This filter is a helper class to apply per band a standard itk::ImageToImageFilter to a VectorImage.
itk::SmartPointer< Self > Pointer
Applies a convolution filter to a mono channel image.
itk::NoiseImageFilter< TPanImageType, InternalImageType > PanNoiseFilterType
FunctorImageFilter< FusionFunctor2 > FusionStep2FilterType
otb::VectorImage< TInternalPrecision, TXsImageType::ImageDimension > InternalVectorImageType
A generic functor filter templated by its functor.
otb::PerBandVectorImageFilter< TXsImageType, InternalVectorImageType, XsConvolutionFilterType > XsVectorConvolutionFilterType
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
Creation of an "otb" image which contains metadata.
itk::SmartPointer< Self > Pointer
itk::SmartPointer< Self > Pointer
InternalImageType::SizeType RadiusType
XsConvolutionFilterType::Pointer m_XsConvolutionFilter
XsVectorNoiseFilterType::Pointer m_XsVectorNoiseFilter
XsVectorConvolutionFilterType::Pointer m_XsVectorConvolutionFilter
void operator()(typename TOutputImageType::PixelType &output, const typename TOutputImageType::PixelType &functor1Pixel, const typename InternalVectorImageType::PixelType &smoothXsPixel, const TInternalPrecision &stdPanchroPixel) const
void operator()(typename TOutputImageType::PixelType &output, const typename InternalVectorImageType::PixelType &stdXsPixel, const TInternalPrecision &smoothPanchroPixel, const typename TPanImageType::PixelType &sharpPanchroPixel) const
LmvmPanSharpeningFusionImageFilter Self
otb::ConvolutionImageFilter< XsBandImageType, InternalImageType, itk::ZeroFluxNeumannBoundaryCondition< TPanImageType >, TInternalPrecision > XsConvolutionFilterType
FusionStep2FilterType::Pointer m_FusionStep2Filter
itk::Array< TInternalPrecision > ArrayType
~LmvmPanSharpeningFusionImageFilter() override
FunctorImageFilter< FusionFunctor1 > FusionStep1FilterType
PanNoiseFilterType::Pointer m_PanNoiseFilter
otb::Image< XsPixelType, TXsImageType::ImageDimension > XsBandImageType
itk::SmartPointer< Self > Pointer
itk::ProgressAccumulator::Pointer m_ProgressAccumulator
This class performs a Local Mean and Variance Matching (LMVM) Pan sharpening operation.
TXsImageType::InternalPixelType XsPixelType
FusionStep1FilterType::Pointer m_FusionStep1Filter
Superclass::PixelType PixelType
otb::Image< TInternalPrecision, TPanImageType::ImageDimension > InternalImageType
constexpr vcl_size_t OutputSize(const std::array< vcl_size_t, 3 > inputsNbBands) const
itk::NoiseImageFilter< XsBandImageType, InternalImageType > XsNoiseFilterType
itk::ImageToImageFilter< TXsImageType, TOutputImageType > Superclass
PanConvolutionFilterType::Pointer m_PanConvolutionFilter
constexpr vcl_size_t OutputSize(const std::array< vcl_size_t, 3 > inputsNbBands) const
XsNoiseFilterType::Pointer m_XsNoiseFilter
otb::PerBandVectorImageFilter< TXsImageType, InternalVectorImageType, XsNoiseFilterType > XsVectorNoiseFilterType
otb::ConvolutionImageFilter< TPanImageType, InternalImageType, itk::ZeroFluxNeumannBoundaryCondition< TPanImageType >, TInternalPrecision > PanConvolutionFilterType
itk::SmartPointer< const Self > ConstPointer
Creation of an "otb" vector image which contains metadata.
Superclass::SizeType SizeType