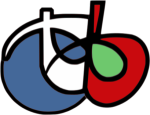 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbFunctorImageFilter_h
22 #define otbFunctorImageFilter_h
27 #include "itkRGBPixel.h"
28 #include "itkRGBAPixel.h"
29 #include "itkFixedArray.h"
30 #include "itkDefaultConvertPixelTraits.h"
31 #include <type_traits>
32 #include "itkConstNeighborhoodIterator.h"
58 struct IsNeighborhood<const
itk::ConstNeighborhoodIterator<VectorImage<T>>&> : std::true_type
86 template <
class T,
unsigned int N>
114 static_assert(
IsSuitableType<T>::value,
"T can not be used as a template parameter for Image or VectorImage classes.");
122 static_assert(
IsSuitableType<T>::value,
"T can not be used as a template parameter for Image or VectorImage classes.");
130 static_assert(
IsSuitableType<T>::value,
"T can not be used as a template parameter for Image or VectorImage classes.");
157 template <
typename T>
158 using RemoveCVRef =
typename std::remove_cv<typename std::remove_reference<T>::type>::type;
168 template <
typename T>
171 static_assert(std::is_class<T>::value || std::is_function<T>::value,
"T is not a class or function");
172 using Type = decltype(&T::operator());
188 template <
typename T,
typename TNameMap>
193 namespace functor_filter_details
195 template <
typename R,
typename TNameMap,
typename... T>
202 template <
typename V>
217 template <
typename R,
typename... T,
typename TNameMap>
226 template <
typename C,
typename R,
typename... T,
typename TNameMap>
235 template <
typename C,
typename R,
typename... T,
typename TNameMap>
244 template <
typename R,
typename... T,
typename TNameMap>
253 template <
typename C,
typename R,
typename... T,
typename TNameMap>
262 template <
typename C,
typename R,
typename... T,
typename TNameMap>
297 template <
typename Functor,
typename TNameMap =
void>
321 template <
class TFunction,
class TNameMap =
void>
344 using Superclass::NumberOfInputs;
350 template <
typename F = TFunction>
351 static std::enable_if_t<std::is_default_constructible<F>::value,
Pointer>
New()
364 template <
typename F = TFunction>
365 static std::enable_if_t<!std::is_default_constructible<F>::value,
Pointer>
New()
367 static_assert(std::is_default_constructible<F>::value,
369 "function as the functor used for the filter creation is not default "
403 void operator=(
const Self&) =
delete;
408 friend auto NewFunctorFilter<TFunction, TNameMap>(TFunction f, itk::Size<2> radius);
411 void ThreadedGenerateData(
const OutputImageRegionType& outputRegionForThread, itk::ThreadIdType threadId)
override;
416 void GenerateInputRequestedRegion(
void)
override;
421 void GenerateOutputInformation()
override;
432 template <
typename Functor,
typename TNameMap>
436 using PointerType =
typename FilterType::Pointer;
438 PointerType p =
new FilterType(f, radius);
454 template <
typename F>
491 template <
typename Functor,
typename TNameMap =
void>
492 auto NewFunctorFilter(Functor f,
unsigned int numberOfOutputBands, itk::Size<2> radius = {{0, 0}})
494 using FunctorType = NumberOfOutputBandsDecorator<Functor>;
495 FunctorType decoratedF(f, numberOfOutputBands);
496 return NewFunctorFilter<FunctorType, TNameMap>(decoratedF, radius);
501 #ifndef OTB_MANUAL_INSTANTIATION
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::OutputImageType OutputImageType
typename Superclass::OutputImageType OutputImageType
Helper struct to check if a type can be used as pixel type.
static std::enable_if_t< std::is_default_constructible< F >::value, Pointer > New()
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::InputHasNeighborhood InputHasNeighborhood
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::InputHasNeighborhood InputHasNeighborhood
constexpr vcl_size_t OutputSize(...) const
const FunctorType & GetFunctor() const
typename std::conditional< std::is_void< TNameMap >::value, VariadicInputsImageFilter< OutputImageType, InputImageType< T >... >, VariadicNamedInputsImageFilter< OutputImageType, TNameMap, InputImageType< T >... > >::type FilterType
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::InputHasNeighborhood InputHasNeighborhood
A generic functor filter templated by its functor.
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
Creation of an "otb" image which contains metadata.
itk::SmartPointer< Self > Pointer
typename OutputImageType::RegionType OutputImageRegionType
itk::SmartPointer< const Self > ConstPointer
std::tuple< typename IsNeighborhood< T >::type... > InputHasNeighborhood
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::InputHasNeighborhood InputHasNeighborhood
constexpr NumberOfOutputBandsDecorator(F t, unsigned int nbComp)
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::OutputImageType OutputImageType
Helper struct to derive PixelType from template parameter.
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::FilterType FilterType
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::FilterType FilterType
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::FilterType FilterType
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::InputHasNeighborhood InputHasNeighborhood
Helper struct to derive ImageType from template parameter.
typename SuperclassHelper::InputHasNeighborhood InputHasNeighborhood
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::OutputImageType OutputImageType
decltype(&T::operator()) Type
itk::VariableLengthVector< T > PixelType
typename SuperclassHelper::FilterType Superclass
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::FilterType FilterType
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::FilterType FilterType
Struct testing if T is a neighborhood.
This struct allows forwarding the operator of template parameter, while adding number of output compo...
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::FilterType FilterType
typename ImageTypeDeduction< typename PixelTypeDeduction< RemoveCVRef< V > >::PixelType >::ImageType InputImageType
typename std::remove_cv< typename std::remove_reference< T >::type >::type RemoveCVRef
that has std::remove_cvref)
typename Superclass::InputTypesTupleType InputTypesTupleType
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::OutputImageType OutputImageType
typename ImageTypeDeduction< R >::ImageType OutputImageType
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::OutputImageType OutputImageType
FunctorType & GetModifiableFunctor()
typename Superclass::template InputImageType< I > InputImageType
Struct to retrieve the operator type.
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::OutputImageType OutputImageType
Struct allowing to derive the superclass prototype for the FunctorImageFilter class.
typename functor_filter_details::FunctorFilterSuperclassHelperImpl< R, TNameMap, T... >::InputHasNeighborhood InputHasNeighborhood
static std::enable_if_t<!std::is_default_constructible< F >::value, Pointer > New()
auto NewFunctorFilter(Functor f, itk::Size< 2 > radius={{0, 0}})
This helper method builds a fully functional FunctorImageFilter from a functor instance.
Creation of an "otb" vector image which contains metadata.
unsigned int m_NumberOfOutputBands
FunctorImageFilter(const FunctorType &f, itk::Size< 2 > radius)
Constructor of functor filter, will copy the functor.