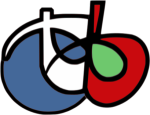 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbParserXPlugins_h
22 #define otbParserXPlugins_h
27 #if defined(__clang__)
28 #pragma clang diagnostic push
30 #if defined(__apple_build_version__)
32 #if __apple_build_version__ >= 7000053
33 #pragma clang diagnostic ignored "-Winconsistent-missing-override"
35 #elif __clang_major__ > 3 || (__clang_major__ == 3 && __clang_minor__ >= 7)
36 #pragma clang diagnostic ignored "-Winconsistent-missing-override"
40 #pragma clang diagnostic pop
52 class bands :
public mup::ICallback
55 bands() : ICallback(mup::cmFUNC,
"bands", 2)
59 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int a_iArgc)
override;
61 const mup::char_type*
GetDesc()
const override
63 return "bands - A bands selector";
66 mup::IToken*
Clone()
const override
68 return new bands(*
this);
73 class dotpr :
public mup::ICallback
76 dotpr() : ICallback(mup::cmFUNC,
"dotpr", -1)
80 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int a_iArgc)
override;
82 const mup::char_type*
GetDesc()
const override
84 return "dotpr(m1,m2) - A vector/matrix dot product";
87 mup::IToken*
Clone()
const override
89 return new dotpr(*
this);
100 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int)
override;
102 const mup::char_type*
GetDesc()
const override
104 return _T(
"x div y - Element-wise division (vectors / matrices)");
121 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int)
override;
123 const mup::char_type*
GetDesc()
const override
125 return _T(
"x dv y - division of vectors / matrices by a scalar");
142 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int)
override;
144 const mup::char_type*
GetDesc()
const override
146 return _T(
"x mult y - Element wise multiplication (vectors / matrices)");
163 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int)
override;
165 const mup::char_type*
GetDesc()
const override
167 return _T(
"x mlt y - multiplication of vectors / matrices by a scalar");
184 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
186 const mup::char_type*
GetDesc()
const override
188 return _T(
"pow - Power for noncomplex vectors & matrices");
205 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int)
override;
207 const mup::char_type*
GetDesc()
const override
209 return _T(
"x pw y - power of vectors / matrices by a scalar");
219 class ndvi :
public mup::ICallback
222 ndvi() : ICallback(mup::cmFUNC,
"ndvi", 2)
226 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int a_iArgc)
override;
228 const mup::char_type*
GetDesc()
const override
230 return "NDVI - Normalized Difference Vegetation Index";
235 return new ndvi(*
this);
240 class cat :
public mup::ICallback
243 cat() : ICallback(mup::cmFUNC,
"cat", -1)
247 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int a_iArgc)
override;
249 const mup::char_type*
GetDesc()
const override
251 return "cat(m1,m2) - Values concatenation";
256 return new cat(*
this);
261 class mean :
public mup::ICallback
264 mean() : ICallback(mup::cmFUNC,
"mean", -1)
268 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int a_iArgc)
override;
270 const mup::char_type*
GetDesc()
const override
272 return "mean(m1,m2,..) - mean of each neighborhood";
277 return new mean(*
this);
282 class var :
public mup::ICallback
285 var() : ICallback(mup::cmFUNC,
"var", -1)
289 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
291 const mup::char_type*
GetDesc()
const override
293 return "var(m1,m2,..) - variance of each neighborhood";
298 return new var(*
this);
303 class corr :
public mup::ICallback
306 corr() : ICallback(mup::cmFUNC,
"corr", 2)
310 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
312 const mup::char_type*
GetDesc()
const override
314 return "corr(m1,m2) - variance of two variables m1 and m2";
319 return new corr(*
this);
327 median() : ICallback(mup::cmFUNC,
"median", -1)
331 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int a_iArgc)
override;
333 const mup::char_type*
GetDesc()
const override
335 return "median(m1,m2,..) - median value of each neighborhood";
345 class maj :
public mup::ICallback
348 maj() : ICallback(mup::cmFUNC,
"maj", -1)
352 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int a_iArgc)
override;
354 const mup::char_type*
GetDesc()
const override
356 return "maj(m1,m2,..) - majority value of each neighborhood";
361 return new maj(*
this);
369 vnorm() : ICallback(mup::cmFUNC,
"vnorm", 1)
373 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int a_iArgc)
override;
375 const mup::char_type*
GetDesc()
const override
377 return "vnorm(v1) - Norm for a vector : sqrt(sum of squared elements); works also with matrices";
382 return new vnorm(*
this);
386 class vmin :
public mup::ICallback
389 vmin() : ICallback(mup::cmFUNC,
"vmin", 1)
393 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
395 const mup::char_type*
GetDesc()
const override
397 return "vmin(m1) - overall minimum";
402 return new vmin(*
this);
407 class vmax :
public mup::ICallback
410 vmax() : ICallback(mup::cmFUNC,
"vmax", 1)
414 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
416 const mup::char_type*
GetDesc()
const override
418 return "vmax(m1) - overall maximum";
423 return new vmax(*
this);
435 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
437 const mup::char_type*
GetDesc()
const override
439 return "vect2scal - Convert one dimensional vector to scalar";
449 class vcos :
public mup::ICallback
452 vcos() : ICallback(mup::cmFUNC,
"vcos", 1)
456 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
458 const mup::char_type*
GetDesc()
const override
460 return "vcos - Cosinus for noncomplex vectors & matrices";
465 return new vcos(*
this);
473 vacos() : ICallback(mup::cmFUNC,
"vacos", 1)
477 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
479 const mup::char_type*
GetDesc()
const override
481 return "vacos - Arccosinus for noncomplex vectors & matrices";
486 return new vacos(*
this);
490 class vsin :
public mup::ICallback
493 vsin() : ICallback(mup::cmFUNC,
"vsin", 1)
497 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
499 const mup::char_type*
GetDesc()
const override
501 return "vsin - Sinus for noncomplex vectors & matrices";
506 return new vsin(*
this);
513 vasin() : ICallback(mup::cmFUNC,
"vasin", 1)
517 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
519 const mup::char_type*
GetDesc()
const override
521 return "vasin - Arcsinus for noncomplex vectors & matrices";
526 return new vasin(*
this);
531 class vtan :
public mup::ICallback
534 vtan() : ICallback(mup::cmFUNC,
"vtan", 1)
538 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
540 const mup::char_type*
GetDesc()
const override
542 return "vtan - Tangent for noncomplex vectors & matrices";
547 return new vtan(*
this);
555 vatan() : ICallback(mup::cmFUNC,
"vatan", 1)
559 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
561 const mup::char_type*
GetDesc()
const override
563 return "vatan - Arctangent for noncomplex vectors & matrices";
568 return new vatan(*
this);
576 vtanh() : ICallback(mup::cmFUNC,
"vtanh", 1)
580 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
582 const mup::char_type*
GetDesc()
const override
584 return "vtanh - Hyperbolic tangent for noncomplex vectors & matrices";
589 return new vtanh(*
this);
597 vsinh() : ICallback(mup::cmFUNC,
"vsinh", 1)
601 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
603 const mup::char_type*
GetDesc()
const override
605 return "vsinh - Hyperbolic sinus for noncomplex vectors & matrices";
610 return new vsinh(*
this);
618 vcosh() : ICallback(mup::cmFUNC,
"vcosh", 1)
622 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
624 const mup::char_type*
GetDesc()
const override
626 return "vcosh - Hyperbolic cosinus for noncomplex vectors & matrices";
631 return new vcosh(*
this);
636 class vlog :
public mup::ICallback
639 vlog() : ICallback(mup::cmFUNC,
"vlog", 1)
643 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
645 const mup::char_type*
GetDesc()
const override
647 return "vlog - Log for noncomplex vectors & matrices";
652 return new vlog(*
this);
660 vlog10() : ICallback(mup::cmFUNC,
"vlog10", 1)
664 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
666 const mup::char_type*
GetDesc()
const override
668 return "vlog10 - Log10 for noncomplex vectors & matrices";
678 class vabs :
public mup::ICallback
681 vabs() : ICallback(mup::cmFUNC,
"vabs", 1)
685 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
687 const mup::char_type*
GetDesc()
const override
689 return "vabs - Absolute value for noncomplex vectors & matrices";
694 return new vabs(*
this);
699 class vexp :
public mup::ICallback
702 vexp() : ICallback(mup::cmFUNC,
"vexp", 1)
706 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
708 const mup::char_type*
GetDesc()
const override
710 return "vexp - Exponential for noncomplex vectors & matrices";
715 return new vexp(*
this);
723 vsqrt() : ICallback(mup::cmFUNC,
"vsqrt", 1)
727 void Eval(mup::ptr_val_type& ret,
const mup::ptr_val_type* a_pArg,
int itkNotUsed(a_iArgc))
override;
729 const mup::char_type*
GetDesc()
const override
731 return "vsqrt - Sqrt for noncomplex vectors & matrices";
736 return new vsqrt(*
this);
mup::IToken * Clone() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int a_iArgc) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
mup::IToken * Clone() const override
const mup::char_type * GetDesc() const override
mup::IToken * Clone() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
const mup::char_type * GetDesc() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
mup::IToken * Clone() const override
mup::IToken * Clone() const override
mup::IToken * Clone() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
mup::IToken * Clone() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
mup::IToken * Clone() const override
mup::IToken * Clone() const override
const mup::char_type * GetDesc() const override
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
const mup::char_type * GetDesc() const override
const mup::char_type * GetDesc() const override
mup::IToken * Clone() const override
const mup::char_type * GetDesc() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
const mup::char_type * GetDesc() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
const mup::char_type * GetDesc() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
mup::IToken * Clone() const override
const mup::char_type * GetDesc() const override
const mup::char_type * GetDesc() const override
const mup::char_type * GetDesc() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int a_iArgc) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int a_iArgc) override
const mup::char_type * GetDesc() const override
mup::IToken * Clone() const override
mup::IToken * Clone() const override
const mup::char_type * GetDesc() const override
const mup::char_type * GetDesc() const override
mup::IToken * Clone() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int a_iArgc) override
const mup::char_type * GetDesc() const override
const mup::char_type * GetDesc() const override
const mup::char_type * GetDesc() const override
const mup::char_type * GetDesc() const override
mup::IToken * Clone() const override
mup::IToken * Clone() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
ElementWiseMultiplication()
mup::IToken * Clone() const override
mup::IToken * Clone() const override
const mup::char_type * GetDesc() const override
mup::IToken * Clone() const override
const mup::char_type * GetDesc() const override
const mup::char_type * GetDesc() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int a_iArgc) override
const mup::char_type * GetDesc() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int a_iArgc) override
mup::IToken * Clone() const override
const mup::char_type * GetDesc() const override
mup::IToken * Clone() const override
mup::IToken * Clone() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int a_iArgc) override
const mup::char_type * GetDesc() const override
const mup::char_type * GetDesc() const override
mup::IToken * Clone() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
mup::IToken * Clone() const override
const mup::char_type * GetDesc() const override
mup::IToken * Clone() const override
const mup::char_type * GetDesc() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
mup::IToken * Clone() const override
const mup::char_type * GetDesc() const override
const mup::char_type * GetDesc() const override
const mup::char_type * GetDesc() const override
mup::IToken * Clone() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
mup::IToken * Clone() const override
void Eval(mup::ptr_val_type &ret, const mup::ptr_val_type *a_pArg, int) override
mup::IToken * Clone() const override
const mup::char_type * GetDesc() const override
const mup::char_type * GetDesc() const override
mup::IToken * Clone() const override
mup::IToken * Clone() const override
mup::IToken * Clone() const override