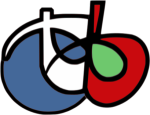 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbAttributesMapLabelObject_h
22 #define otbAttributesMapLabelObject_h
24 #if defined(__GNUC__) || defined(__clang__)
25 #pragma GCC diagnostic push
26 #pragma GCC diagnostic ignored "-Wunused-parameter"
27 #include "itkShapeLabelObject.h"
28 #pragma GCC diagnostic pop
30 #include "itkShapeLabelObject.h"
53 template <
class TLabelObject>
68 return labelObject->GetAttribute(m_AttributeName.c_str());
74 m_AttributeName = name;
79 return m_AttributeName.c_str();
105 template <
class TLabelObject,
class TMeasurementVector>
111 inline TMeasurementVector
operator()(
const TLabelObject*
object)
const
115 unsigned int attrIndex = 0;
116 typename AttributesListType::const_iterator attrIt =
m_Attributes.begin();
119 newSample[attrIndex] =
object->GetAttribute(attrIt->c_str());
176 template <
class TLabel,
unsigned int VImageDimension,
class TAttributesValue>
182 typedef itk::LabelObject<TLabel, VImageDimension>
Superclass;
194 itkStaticConstMacro(ImageDimension,
unsigned int, VImageDimension);
223 m_Attributes[name] = value;
232 this->SetAttribute(name.c_str(), value);
241 if (it != m_Attributes.end())
247 itkExceptionMacro(<<
"Could not find attribute named " << name);
257 return m_Attributes.size();
265 std::vector<std::string> attributesNames;
269 while (it != m_Attributes.end())
271 attributesNames.push_back(it->first);
274 return attributesNames;
282 Superclass::CopyAttributesFrom(lo);
285 const Self* src =
dynamic_cast<const Self*
>(lo);
323 void PrintSelf(std::ostream& os, itk::Indent indent)
const override
325 Superclass::PrintSelf(os, indent);
326 os << indent <<
"Attributes: " << std::endl;
329 os << indent << indent << it->first <<
" = " << it->second << std::endl;
336 void operator=(
const Self&) =
delete;
std::vector< std::string > GetAvailableAttributes() const
const PolygonType * GetPolygon() const
std::map< std::string, AttributesValueType > AttributesMapType
Map container typedefs.
AttributesMapType m_Attributes
~AttributesMapLabelObjectAccessor()
Destructor.
std::string m_AttributeName
Name of the attribute to retrieve.
void RemoveAttribute(const char *attr)
Superclass::LabelObjectType LabelObjectType
A LabelObject with a generic attributes map.
AttributesListType m_Attributes
itk::LabelObject< TLabel, VImageDimension > Superclass
AttributesMapType::const_iterator AttributesMapConstIteratorType
string_view find(string_view const &haystack, string_view const &needle)
itk::LabelMap< Self > LabelMapType
Type of a label map using an AttributesMapLabelObject.
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
const AttributeValueType operator()(LabelObjectType *labelObject) const
void SetAttribute(const char *name, AttributesValueType value)
TLabelObject LabelObjectType
void PrintSelf(std::ostream &os, itk::Indent indent) const override
itk::SmartPointer< Self > Pointer
AttributesMapType::iterator AttributesMapIteratorType
TMeasurementVector operator()(const TLabelObject *object) const
Superclass::LineType LineType
TAttributesValue AttributesValueType
virtual void CopyAttributesFrom(const LabelObjectType *lo)
AttributesMapLabelObjectAccessor()
Constructor.
AttributesValueType GetAttribute(const char *name) const
std::vector< std::string > AttributesListType
TLabel LabelType
Template parameters typedef.
Allows accessing a given field of an AttributesMapLabelObject.
This class allows building a measurement vector from an AttributesMapLabelObject.
Polygon< double > PolygonType
LabelObjectType::AttributesValueType AttributeValueType
void SetAttribute(const std::string &name, AttributesValueType value)
PolygonType::Pointer PolygonPointerType
~AttributesMapLabelObject() override
unsigned int GetNumberOfAttributes() const
itk::SmartPointer< Self > Pointer
void SetAttributeName(const char *name)
Set the name of the attribute to retrieve.
const char * GetAttributeName() const
Get the the name of the attribute to retrieve.
Superclass::IndexType IndexType
PolygonType * GetPolygon()
unsigned int GetNumberOfAttributes()
This class represent a 2D polygon.
void AddAttribute(const char *attr)
void SetPolygon(PolygonType *p)
AttributesMapLabelObject Self
Superclass::LengthType LengthType
AttributesMapLabelObject()
itk::SmartPointer< const Self > ConstPointer
itk::WeakPointer< const Self > ConstWeakPointer
PolygonPointerType m_Polygon