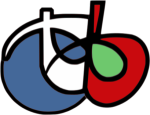 |
OTB
9.1.1
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbStringUtilities_h
22 #define otbStringUtilities_h
36 #include "OTBCommonExport.h"
129 template <std::
size_t N>
144 assert(first <= last);
151 template <
typename II>
159 template <
typename II>
163 assert(first <= last);
174 return first <= &*
begin() && &*
end() <= last;
176 template <std::
size_t N>
182 return belongs_to(&ptr[0], &ptr[std::strlen(ptr)]);
184 template <
typename String>
186 return belongs_to(s.data(), s.data() + s.size());
262 return ! (lhs == rhs);
268 std::copy(v.
begin(), v.
end(), std::ostream_iterator<char>(os));
275 std::string res(lhs.
begin(), lhs.
end());
291 return needle.
size() <= haystack.
size()
292 && std::equal(needle.
begin(), needle.
end(), haystack.
begin());
299 return needle.
size() <= haystack.
size()
307 assert(!haystack.
empty());
308 assert(!needle.
empty());
310 if (haystack.
size() < size) {
319 for ( ; it != end ; ++it) {
321 assert(v.
size() == size);
331 assert(it+size-1 == haystack.
end());
354 template <
typename Splitter>
404 return ! (lhs == rhs);
406 #if defined(BOOST_TEST_MODULE)
409 return os <<
"split iterator on `" << v.
m_crt
410 <<
"'("<<
static_cast<void const*
>(&*v.
m_crt.
begin()) <<
"-"<<v.
m_crt.
size()<<
")"<<
", within `"
421 inline std::size_t
len(
char) {
return 1; }
422 template <std::
size_t N>
inline std::size_t
len(
char const (&)[N])
423 { assert(N>0);
return N-1; }
424 inline std::size_t
len(
char const* ptr) {
return std::strlen(ptr); }
425 template <
typename String>
inline std::size_t
len(String
const& s) {
return s.length(); }
434 template <
typename Splitter>
488 template <
typename String>
517 template <
typename T>
522 std::stringstream ss;
523 if (ss << v && (ss >> res >> std::ws).eof()) {
526 throw std::runtime_error(
"Cannot decode \""+v+
"\" as \""+
527 typeid(T).name() +
"\" while " + context);
548 template <
typename T>
553 std::stringstream ss;
554 if (ss << v && (ss >> res >> std::ws).eof()) {
584 bool is_negative =
false;
588 case '-': is_negative =
true;
592 for ( ; it != end ; ++it) {
594 if (!std::isdigit(*it)) {
595 throw std::runtime_error(
"Cannot decode "+v+
" as integer while " + context);
597 res = 10 * res + *it -
'0';
600 return is_negative ? -res : res;
625 bool is_negative =
false;
629 case '-': is_negative =
true;
632 for ( ; it != end ; ++it) {
634 if (!std::isdigit(*it)) {
637 res = 10 * res + *it -
'0';
640 return is_negative ? -res : res;
665 for ( ; it != end ; ++it) {
667 if (!std::isdigit(*it)) {
668 throw std::runtime_error(
"Cannot decode "+v+
" as integer while " + context);
670 res = 10 * res + *it -
'0';
700 for ( ; it != end ; ++it) {
702 if (!std::isdigit(*it)) {
705 res = 10 * res + *it -
'0';
728 FloatType res = FloatType();
730 std::stringstream ss;
731 if (! (ss << v && (ss >> res >> std::ws).eof())) {
732 throw std::runtime_error(
"Cannot decode "+v+
" as float value while " + context);
759 FloatType res = FloatType();
761 std::stringstream ss;
762 if (! (ss << v && (ss >> res >> std::ws).eof())) {
772 unsigned int res = 0;
776 if (!std::isdigit(v.
front()))
778 res = 10 * res + v.
front() -
'0';
784 #define OTB_GENERATE_CONV(internal_to, type) \
785 template <> inline type to<type>(string_view const& v, string_view const& context) \
786 { return details::internal_to<type>(v, context); } \
787 template <> inline type to_with_default<type>(string_view const& v, type const& def) \
788 { return details::internal_to<type>(v, def); }
801 #if defined(HAS_LONG_LONG) // TODO: add this configure option
808 #undef OTB_GENERATE_CONV
811 {
return std::string(v.
begin(), v.
end()); }
813 template <
typename T>
inline T
const&
to(T
const& v,
string_view const& ) {
return v; }
815 template <
typename T>
inline T
const&
to_with_default(T
const& v, T
const& ) {
return v; }
840 OTBCommon_EXPORT string_view
lstrip(string_view
const& v, string_view
const& c );
848 OTBCommon_EXPORT string_view
rstrip(string_view
const& v, string_view
const& c );
void remove_prefix(std::vcl_size_t n)
Int to_uinteger(string_view const &v, Int const def)
Internal generic string to unsigned integer conversion (w/o exception). Tries to convert the input st...
char_iterator & operator-=(difference_type off)
const_pointer operator->() const
static string_view null()
part_range< splitter_on_delim > split_on(String const &str, char delim)
std::reverse_iterator< char_iterator > const_reverse_iterator
friend char_iterator operator+(difference_type off, char_iterator rhs)
friend char_iterator operator-(char_iterator lhs, difference_type off)
bool belongs_to(char const *ptr) const
char_iterator & operator+=(difference_type off)
std::forward_iterator_tag iterator_category
std::string operator+(string_view const &lhs, string_view const &rhs)
const_reference operator*() const
string_view find(string_view const &haystack, string_view const &needle)
char_iterator operator++(int)
string_view(II first, size_type size_)
std::random_access_iterator_tag iterator_category
const_reverse_iterator crbegin() const
OTBCommon_EXPORT string_view lstrip(string_view const &v, string_view const &c)
returns a string_view with the leading characters removed
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
part_iterator< Splitter > const_iterator
string_view(II first, II last)
char const * const_pointer
string_view(std::string const &s)
constexpr bool operator==(extents< StaticExtentsL... > const &lhs, extents< StaticExtentsR... > const &rhs)
bool starts_with(string_view const &haystack, string_view const &needle)
bool belongs_to(char const (&array)[N]) const
part_iterator & operator++()
std::vcl_size_t size_type
const_iterator cend() const
std::vcl_size_t len(char)
part_iterator(string_view const &global_string, Splitter s)
char_iterator const_iterator
char_iterator(const_pointer p)
const_reverse_iterator rbegin() const
T to_with_default(string_view const &v, T const &def=T())
Generic string to whatever conversion – failure is hidden. Tries to decode a value from a string.
bool belongs_to(char const *first, char const *last) const
const const_iterator m_last
friend char_iterator operator+(char_iterator lhs, difference_type off)
const_iterator cbegin() const
part_range(string_view const &global_string, Splitter s)
FloatType to_float(string_view const &v, string_view const &context)
Internal generic string to float conversion (w/ exception). Tries to convert the input string into a ...
string_view const * const_pointer
const_reference operator*() const
std::vcl_size_t next_start() const
const_pointer data() const
OTBCommon_EXPORT string_view rstrip(string_view const &v, string_view const &c)
returns a string_view with the ending characters removed
bool belongs_to(String const &s) const
Int to_integer(string_view const &v, Int const def)
Internal generic string to integer conversion (w/o exception). Tries to convert the input string into...
const_reference back() const
string_view const & const_reference
friend char_iterator operator-(difference_type off, char_iterator rhs)
T to(string_view const &v, string_view const &context)
Generic string to whatever conversion – failure means exception. Tries to decode a value from a strin...
const_iterator const & begin() const
void remove_suffix(std::vcl_size_t n)
string_view(char const (&array)[N])
part_iterator operator++(int)
const_iterator const & end() const
std::ptrdiff_t difference_type
const_iterator end() const
constexpr bool operator!=(extents< StaticExtentsL... > const &lhs, extents< StaticExtentsR... > const &rhs)
friend bool operator==(part_iterator const &lhs, part_iterator const &rhs)
char const & const_reference
const_iterator begin() const
const_reference front() const
bool ends_with(string_view const &haystack, string_view const &needle)
friend difference_type operator-(char_iterator lhs, char_iterator rhs)
Int to_integer(string_view const &v, string_view const &context)
Internal generic string to integer conversion (w/ exception). Tries to convert the input string into ...
splitter_on_delim(char delim)
const const_iterator m_first
friend bool operator!=(char_iterator lhs, char_iterator rhs)
OTBCommon_EXPORT std::ostream & operator<<(std::ostream &os, const otb::StringToHTML &str)
const_reference operator[](size_type p)
string_view(char const *ptr)
OTB_GENERATE_CONV(to_integer, char)
Generic string to whatever conversion – failure means exception. Tries to decode a value from a strin...
part_iterator(string_view const &global_string, Splitter s, theend)
const_reverse_iterator crend() const
char_iterator operator--(int)
char_iterator & operator++()
friend bool operator==(char_iterator lhs, char_iterator rhs)
const_reverse_iterator rend() const
unsigned int decode_uint(string_view &v)
Internal generic string to float conversion (w/ exception). Tries to convert the input string into a ...
Int to_uinteger(string_view const &v, string_view const &context)
Internal generic string to unsigned integer conversion (w/ exception). Tries to convert the input str...
string_view operator()(string_view const &within) const
friend bool operator<(char_iterator lhs, char_iterator rhs)
bool is_same_view(string_view const &lhs, string_view const &rhs)
FloatType to_float(string_view const &v, FloatType const def)
Internal generic string to float conversion (w/o exception). Tries to convert the input string into a...
friend bool operator<=(char_iterator lhs, char_iterator rhs)
friend bool operator!=(part_iterator const &lhs, part_iterator const &rhs)
std::vcl_size_t size_type
bool contains(string_view const &haystack, string_view const &needle)
char_iterator & operator--()