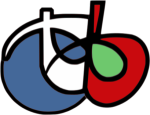 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
22 #ifndef otbStreamingMinMaxVectorImageFilter_h
23 #define otbStreamingMinMaxVectorImageFilter_h
27 #include "itkSimpleDataObjectDecorator.h"
28 #include "itkImageRegionSplitter.h"
29 #include "itkVariableSizeMatrix.h"
30 #include "itkVariableLengthVector.h"
53 template <
class TInputImage>
73 typedef typename TInputImage::SizeType
SizeType;
78 itkStaticConstMacro(InputImageDimension,
unsigned int, TInputImage::ImageDimension);
81 itkStaticConstMacro(ImageDimension,
unsigned int, TInputImage::ImageDimension);
84 typedef typename itk::NumericTraits<InternalPixelType>::RealType
RealType;
92 typedef typename itk::VariableSizeMatrix<RealType>
MatrixType;
114 itkSetMacro(NoDataFlag,
bool);
119 itkGetMacro(NoDataFlag,
bool);
124 itkBooleanMacro(NoDataFlag);
130 return this->GetMinimumOutput()->Get();
132 PixelObjectType* GetMinimumOutput();
133 const PixelObjectType* GetMinimumOutput()
const;
139 return this->GetMaximumOutput()->Get();
141 PixelObjectType* GetMaximumOutput();
142 const PixelObjectType* GetMaximumOutput()
const;
148 DataObjectPointer MakeOutput(DataObjectPointerArraySizeType idx)
override;
149 using Superclass::MakeOutput;
154 void AllocateOutputs()
override;
155 void GenerateOutputInformation()
override;
156 void Synthetize(
void)
override;
157 void Reset(
void)
override;
165 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
167 void ThreadedGenerateData(
const RegionType& outputRegionForThread, itk::ThreadIdType threadId)
override;
171 void operator=(
const Self&) =
delete;
202 template <
class TInputImage>
234 using Superclass::SetInput;
237 this->GetFilter()->SetInput(input);
241 return this->GetFilter()->GetInput();
247 return this->GetFilter()->GetMinimumOutput()->Get();
251 return this->GetFilter()->GetMinimumOutput();
255 return this->GetFilter()->GetMinimumOutput();
262 return this->GetFilter()->GetMaximumOutput()->Get();
266 return this->GetFilter()->GetMaximumOutput();
270 return this->GetFilter()->GetMaximumOutput();
285 void operator=(
const Self&) =
delete;
290 #ifndef OTB_MANUAL_INSTANTIATION
StreamingMinMaxVectorImageFilter()
StatFilterType::RealPixelType RealPixelType
PixelType GetMaximum() const
itk::VariableLengthVector< RealType > RealPixelType
StatFilterType::ArrayLongPixelType ArrayLongPixelType
StreamingMinMaxVectorImageFilter Self
This class streams the whole input image through the PersistentMinMaxVectorImageFilter.
Superclass::FilterType StatFilterType
StatFilterType::MatrixObjectType MatrixObjectType
This filter is the base class for all filter persisting data through multiple update....
Compute min. max of a large image using streaming.
itk::SmartPointer< const Self > ConstPointer
TInputImage::RegionType RegionType
const InputImageType * GetInput()
itk::ProcessObject::DataObjectPointerArraySizeType DataObjectPointerArraySizeType
StatFilterType::ArrayMatrixType ArrayMatrixType
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
StatFilterType::PixelType PixelType
TInputImage InputImageType
const PixelObjectType * GetMinimumOutput() const
TInputImage::SizeType SizeType
const PixelObjectType * GetMaximumOutput() const
itk::SimpleDataObjectDecorator< PixelType > PixelObjectType
ArrayPixelType m_ThreadMax
TInputImage::InternalPixelType InternalPixelType
std::vector< RealPixelType > ArrayRealPixelType
TInputImage::Pointer InputImagePointer
PixelObjectType * GetMinimumOutput()
itk::SmartPointer< const Self > ConstPointer
PixelType GetMinimum() const
itk::SmartPointer< Self > Pointer
~StreamingMinMaxVectorImageFilter() override
StatFilterType::ArrayPixelType ArrayPixelType
StatFilterType::RealType RealType
StatFilterType::PixelObjectType PixelObjectType
ArrayPixelType m_ThreadMin
itk::NumericTraits< InternalPixelType >::RealType RealType
StatFilterType::RealPixelObjectType RealPixelObjectType
PersistentMinMaxVectorImageFilter Self
This filter link a persistent filter with a StreamingImageVirtualWriter.
InternalPixelType m_NoDataValue
PixelType GetMaximum() const
PersistentImageFilter< TInputImage, TInputImage > Superclass
itk::SimpleDataObjectDecorator< RealPixelType > RealPixelObjectType
itk::Array< long > ArrayLongPixelType
PixelObjectType * GetMaximumOutput()
itk::DataObject::Pointer DataObjectPointer
StatFilterType::ArrayRealPixelType ArrayRealPixelType
void SetInput(InputImageType *input)
itk::SmartPointer< Self > Pointer
std::vector< PixelType > ArrayPixelType
itk::SimpleDataObjectDecorator< MatrixType > MatrixObjectType
itk::VariableSizeMatrix< RealType > MatrixType
TInputImage::PixelType PixelType
PersistentFilterStreamingDecorator< PersistentMinMaxVectorImageFilter< TInputImage > > Superclass
PixelType GetMinimum() const
std::vector< MatrixType > ArrayMatrixType
~PersistentMinMaxVectorImageFilter() override
TInputImage::IndexType IndexType
StatFilterType::MatrixType MatrixType