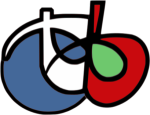 |
OTB
9.1.1
Orfeo Toolbox
|
Go to the documentation of this file.
22 #ifndef otbStatisticsAttributesLabelMapFilter_h
23 #define otbStatisticsAttributesLabelMapFilter_h
26 #include "itkMatrix.h"
27 #include "itkVector.h"
41 template <
class TLabelObject,
class TFeatureImage>
49 typedef typename itk::Matrix<double, TFeatureImage::ImageDimension, TFeatureImage::ImageDimension>
MatrixType;
51 typedef typename itk::Vector<double, TFeatureImage::ImageDimension>
VectorType;
127 template <
class TImage,
class TFeatureImage>
130 typename Functor::StatisticsAttributesLabelObjectFunctor<typename TImage::LabelObjectType, TFeatureImage>>
148 itkStaticConstMacro(ImageDimension,
unsigned int, TImage::ImageDimension);
157 void SetFeatureImage(
const TFeatureImage* input);
163 void SetInput1(
const TImage* input);
166 const TImage* GetInput1()
const;
169 void SetInput2(
const TFeatureImage* input);
172 const TFeatureImage* GetInput2()
const;
175 void SetFeatureName(
const std::string& name);
178 const std::string& GetFeatureName()
const;
181 void SetReducedAttributeSet(
bool flag);
184 bool GetReducedAttributeSet()
const;
186 itkBooleanMacro(ReducedAttributeSet);
196 void BeforeThreadedGenerateData()
override;
199 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
203 void operator=(
const Self&) =
delete;
208 #ifndef OTB_MANUAL_INSTANTIATION
StatisticsAttributesLabelMapFilter Self
virtual ~StatisticsAttributesLabelObjectFunctor()
StatisticsAttributesLabelObjectFunctor Self
std::string m_FeatureName
ImageType::LabelObjectType LabelObjectType
bool operator==(const Self &self)
This class applies a functor to compute new features.
const std::string & GetFeatureName() const
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
TLabelObject LabelObjectType
Typedef of the label object.
TFeatureImage::PixelType FeatureType
Typedef of the feature image type.
void SetReducedAttributeSet(bool flag)
LabelMapFeaturesFunctorImageFilter< ImageType, FunctorType > Superclass
Functor::StatisticsAttributesLabelObjectFunctor< LabelObjectType, FeatureImageType > FunctorType
bool m_ReducedAttributeSet
itk::SmartPointer< Self > Pointer
void SetFeatureName(const std::string &name)
StatisticsAttributesLabelObjectFunctor()
TFeatureImage FeatureImageType
bool GetReducedAttributeSet() const
void SetFeatureImage(const TFeatureImage *img)
bool operator!=(const Self &self)
itk::Vector< double, TFeatureImage::ImageDimension > VectorType
LabelObjectType::ConstLineIterator ConstLineIteratorType
TFeatureImage::ConstPointer m_FeatureImage
const TFeatureImage * GetFeatureImage() const
This class is a fork of itk::StasticsLabelMapFilter to support AttributesMapLabelObject.
itk::Matrix< double, TFeatureImage::ImageDimension, TFeatureImage::ImageDimension > MatrixType
void operator()(LabelObjectType *lo) const
itk::SmartPointer< const Self > ConstPointer
Functor to compute statistics attributes of one LabelObject.