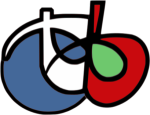 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
22 #ifndef otbShiftScaleImageAdaptor_h
23 #define otbShiftScaleImageAdaptor_h
25 #include "vnl/vnl_math.h"
26 #include "itkImageAdaptor.h"
49 template <
class TPixelType,
class TExternalType>
59 typedef typename itk::NumericTraits<TPixelType>::RealType
InternalType;
106 template <
class TImage,
class TOutputPixelType>
107 class ITK_EXPORT
ShiftScaleImageAdaptor :
public itk::ImageAdaptor<TImage, Accessor::ShiftScalePixelAccessor<typename TImage::PixelType, TOutputPixelType>>
112 typedef itk::ImageAdaptor<TImage, Accessor::ShiftScalePixelAccessor<typename TImage::PixelType, TOutputPixelType>>
Superclass;
128 itkDebugMacro(
"returning "
129 <<
" m_Shift of " << this->GetPixelAccessor().GetShift());
130 return this->GetPixelAccessor().GetShift();
133 virtual void SetShift(
typename TImage::PixelType value)
135 itkDebugMacro(
"setting m_Shift to " << value);
136 if (this->GetPixelAccessor().GetShift() != value)
138 this->GetPixelAccessor().SetShift(value);
145 itkDebugMacro(
"returning "
146 <<
" m_Scale of " << this->GetPixelAccessor().GetScale());
147 return this->GetPixelAccessor().GetScale();
150 virtual void SetScale(
typename TImage::PixelType value)
152 itkDebugMacro(
"setting m_Scale to " << value);
153 if (this->GetPixelAccessor().GetScale() != value)
155 this->GetPixelAccessor().SetScale(value);
170 void operator=(
const Self&) =
delete;
Apply a shift scale operation to the value.
ShiftScaleImageAdaptor Self
void Set(InternalType &output, const ExternalType &input)
Superclass::IndexType IndexType
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
TImage::PixelType GetShift() const
InternalType GetShift() const
virtual void SetShift(typename TImage::PixelType value)
itk::ImageAdaptor< TImage, Accessor::ShiftScalePixelAccessor< typename TImage::PixelType, TOutputPixelType > > Superclass
TImage::PixelType InternalType
itk::NumericTraits< TPixelType >::RealType InternalType
void SetShift(InternalType value)
Accessor::ShiftScalePixelAccessor< typename TImage::PixelType, TOutputPixelType > AccessorType
~ShiftScaleImageAdaptor() override
void SetScale(InternalType value)
TImage::PixelType GetScale() const
const ExternalType Get(const InternalType &input) const
itk::SmartPointer< const Self > ConstPointer
itk::SmartPointer< Self > Pointer
virtual void SetScale(typename TImage::PixelType value)
Presents an image as being composed of the shift scale operation of its pixels.
TExternalType ExternalType
AccessorType::ExternalType PixelType
InternalType GetScale() const