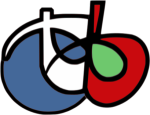 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
22 #ifndef otbShapeAttributesLabelMapFilter_h
23 #define otbShapeAttributesLabelMapFilter_h
45 template <
class TLabelObject,
class TLabelImage>
70 itkStaticConstMacro(
ImageDimension,
unsigned int, TLabelObject::ImageDimension);
71 typedef itk::ImageRegion<TLabelObject::ImageDimension>
RegionType;
72 typedef itk::Offset<TLabelObject::ImageDimension>
OffsetType;
154 typedef std::map<Offset2Type, itk::SizeValueType, Offset2Type::LexicographicCompare>
MapIntercept2Type;
155 typedef std::map<Offset3Type, itk::SizeValueType, Offset3Type::LexicographicCompare>
MapIntercept3Type;
157 template <
class TMapIntercept,
class TSpacing>
160 #if !defined(ITK_DO_NOT_USE_PERIMETER_SPECIALIZATION)
211 template <
class TImage,
class TLabelImage = Image<
typename TImage::PixelType, TImage::ImageDimension>>
232 itkStaticConstMacro(ImageDimension,
unsigned int, TImage::ImageDimension);
244 void SetComputeFeretDiameter(
bool flag);
245 bool GetComputeFeretDiameter()
const;
246 itkBooleanMacro(ComputeFeretDiameter);
253 void SetComputePerimeter(
bool flag);
254 bool GetComputePerimeter()
const;
255 itkBooleanMacro(ComputePerimeter);
262 void SetComputePolygon(
bool flag);
263 bool GetComputePolygon()
const;
264 itkBooleanMacro(ComputePolygon);
271 void SetComputeFlusser(
bool flag);
272 bool GetComputeFlusser()
const;
273 itkBooleanMacro(ComputeFlusser);
278 void SetReducedAttributeSet(
bool flag);
279 bool GetReducedAttributeSet()
const;
280 itkBooleanMacro(ReducedAttributeSet);
285 void SetLabelImage(
const TLabelImage*);
286 const TLabelImage* GetLabelImage()
const;
301 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
303 void AllocateOutputs()
override;
306 void BeforeThreadedGenerateData()
override;
308 void GenerateInputRequestedRegion()
override;
314 void operator=(
const Self&) =
delete;
320 #ifndef OTB_MANUAL_INSTANTIATION
This class is a fork of the itk::ShapeLabelMapFilter working with AttributesMapLabelObject.
TLabelObject::PolygonType PolygonType
double PerimeterFromInterceptCount(TMapIntercept &intercepts, const TSpacing &spacing)
This filter performs a simplification of the input path.
Calculate the Flusser's invariant parameters.
This class vectorizes a LabelObject to a Polygon.
unsigned int DimensionType
bool operator!=(const Self &self)
bool GetComputeFlusser() const
This class applies a functor to compute new features.
static double hyperSpherePerimeter(double radius)
itk::Offset< TLabelObject::ImageDimension > OffsetType
bool GetReducedAttributeSet() const
void EnlargeOutputRequestedRegion(itk::DataObject *) override
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
std::map< Offset3Type, itk::SizeValueType, Offset3Type::LexicographicCompare > MapIntercept3Type
void SetReducedAttributeSet(bool flag)
std::map< Offset2Type, itk::SizeValueType, Offset2Type::LexicographicCompare > MapIntercept2Type
void SetLabelImage(const TLabelImage *image)
bool GetComputeFeretDiameter() const
bool GetComputePerimeter() const
SimplifyPathFunctor< PolygonType, PolygonType > SimplifyPolygonFunctorType
void SetComputeFeretDiameter(bool flag)
static double gammaN2p1(long n)
itk::Vector< double, 2 > Spacing2Type
~ShapeAttributesLabelMapFilter() override
void operator()(LabelObjectType *lo)
const TLabelImage * GetLabelImage() const
static const unsigned int ImageDimension
LabelObjectType::ConstLineIterator ConstLineIteratorType
LabelImageType::ConstPointer m_LabelImage
Functor::LabelObjectToPolygonFunctor< LabelObjectType, PolygonType > PolygonFunctorType
double ComputePerimeter(LabelObjectType *labelObject, const RegionType ®ion)
ShapeAttributesLabelMapFilter Self
Functor::ShapeAttributesLabelObjectFunctor< LabelObjectType, LabelImageType > FunctorType
TLabelImage LabelImageType
void SetComputeFlusser(bool flag)
static long factorial(long n)
TLabelObject LabelObjectType
itk::Vector< double, 3 > Spacing3Type
itk::Offset< 3 > Offset3Type
virtual ~ShapeAttributesLabelObjectFunctor()
ShapeAttributesLabelObjectFunctor Self
static long doubleFactorial(long n)
Functor to compute shape attributes of one LabelObject.
itk::SmartPointer< const Self > ConstPointer
void SetComputePolygon(bool flag)
LabelMapFeaturesFunctorImageFilter< ImageType, FunctorType > Superclass
itk::ImageRegion< TLabelObject::ImageDimension > RegionType
itk::Offset< 2 > Offset2Type
ShapeAttributesLabelObjectFunctor()
bool operator==(const Self &self)
static double hyperSphereRadiusFromVolume(double volume)
itk::SmartPointer< Self > Pointer
FlusserPathFunction< PolygonType > FlusserPathFunctionType
bool m_ReducedAttributeSet
bool GetComputePolygon() const
static double hyperSphereVolume(double radius)
ImageType::Pointer ImagePointer
ImageType::RegionType InputImageRegionType
void SetComputePerimeter(bool flag)
ImageType::LabelObjectType LabelObjectType
TLabelImage LabelImageType
ShapeAttributesLabelMapFilter()
bool m_ComputeFeretDiameter