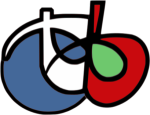 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
23 #ifndef otbPrintableImageFilter_h
24 #define otbPrintableImageFilter_h
27 #include "itkImageToImageFilter.h"
30 #include "itkBinaryFunctorImageFilter.h"
44 template <
class TInputPixel,
class TMaskPixel,
class TOutputPixel>
50 m_BackgroundValue = 0;
51 m_ObjectColor.SetSize(3);
52 m_ObjectColor.Fill(255);
65 return m_BackgroundValue;
69 m_BackgroundValue = val;
84 if (maskPix == m_BackgroundValue)
86 outPix.SetSize(inPix.Size());
87 for (
unsigned int i = 0; i < outPix.Size(); ++i)
94 outPix = m_ObjectColor;
123 template <
class TInputImage,
class TMaskImage = otb::Image<
unsigned char, 2>>
124 class ITK_EXPORT
PrintableImageFilter :
public itk::ImageToImageFilter<TInputImage, otb::VectorImage<unsigned char, 2>>
150 typedef itk::BinaryFunctorImageFilter<OutputImageType, MaskImageType, OutputImageType, FunctorType>
FunctorFilterType;
160 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
162 void SetChannel(
unsigned int channel);
184 itkSetMacro(UseMask,
bool);
185 itkGetMacro(UseMask,
bool);
189 return m_ChannelList;
194 if (chList.size() != 3)
196 itkExceptionMacro(<<
"Invalid channel list, size is " << chList.size() <<
" instead of 3");
198 m_ChannelList = chList;
205 if (val.GetSize() != 3)
207 itkExceptionMacro(<<
"Invalid object color, size is " << val.Size() <<
" instead of 3");
210 m_MaskFilter->GetFunctor().SetObjectColor(val);
213 itkGetMacro(ObjectColor, OutputPixelType);
218 m_BackgroundMaskValue = val;
219 m_MaskFilter->GetFunctor().SetBackgroundValue(val);
222 itkGetMacro(BackgroundMaskValue, MaskPixelType);
232 void GenerateOutputInformation()
override;
237 void BeforeGenerateData();
238 void GenerateData()
override;
242 void operator=(
const Self&);
262 #ifndef OTB_MANUAL_INSTANTIATION
FunctorFilterPointerType m_MaskFilter
VectorImage< OutputInternalPixelType, 2 > OutputImageType
MaskImageType::PixelType MaskPixelType
const ChannelsType GetChannelList()
OutputPixelType operator()(InputPixelType inPix, MaskPixelType maskPix) const
void SetObjectColor(OutputPixelType val)
This filter performs a rescaling of a vector image on a per band basis.
void SetBackgroundMaskValue(MaskPixelType val)
void SetChannelList(ChannelsType chList)
template class OTBImageBase_EXPORT_TEMPLATE VectorImage< unsigned char, 2 >
OutputPixelType::ValueType OutputInternalPixelType
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
InputImageType::PixelType InputPixelType
itk::SmartPointer< const Self > ConstPointer
PrintableImageFilter Self
ChannelsType m_ChannelList
otb::MultiChannelExtractROI< InputInternalPixelType, InputInternalPixelType > ChannelExtractorType
void SetBackgroundValue(MaskPixelType val)
Output is a InputPixel if MaskPixel is m_Background and a defined other value (m_ObjectColor) otherwi...
void SetObjectColor(OutputPixelType val)
MaskPixelType m_BackgroundMaskValue
MaskImageType::Pointer MaskImagePointerType
OutputPixelType m_ObjectColor
OutputPixelType m_ObjectColor
VectorRescalerType::Pointer m_Rescaler
OutputPixelType GetObjectColor()
TInputPixel InputPixelType
itk::SmartPointer< Self > Pointer
VectorRescaleIntensityImageFilter< typename ChannelExtractorType::OutputImageType, OutputImageType > VectorRescalerType
InputImageType::InternalPixelType InputInternalPixelType
TOutputPixel OutputPixelType
FunctorFilterType::Pointer FunctorFilterPointerType
ChannelExtractorType::Pointer m_Extractor
Superclass::PixelType PixelType
ChannelExtractorType::ChannelsType ChannelsType
MaskPixelType m_BackgroundValue
itk::SmartPointer< Self > Pointer
itk::ImageToImageFilter< TInputImage, otb::VectorImage< unsigned char, 2 > > Superclass
OutputImageType::PixelType OutputPixelType
MaskPixelType GetBackgroundValue()
TInputImage InputImageType
#define otbGetObjectMemberMacro(object, name, type)
unsigned char OutputInternalPixelType
#define otbSetObjectMemberMacro(object, name, type)
Functor::MaskFunctor< InputPixelType, MaskPixelType, OutputPixelType > FunctorType
itk::BinaryFunctorImageFilter< OutputImageType, MaskImageType, OutputImageType, FunctorType > FunctorFilterType
This class is a helper class to turn a vector image to a generic 8 bytes RGB image....
Creation of an "otb" vector image which contains metadata.