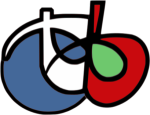 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
26 #include <type_traits>
38 template<
class ElementType,
49 using mapping_type =
typename layout_type::template mapping<extents_type>;
67 template<class... IndexType>
80 template<
class... IndexType>
88 template<
class IndexType,
size_t N>
99 template<
class OtherElementType,
class OtherExtents,
class OtherLayoutPolicy>
109 template<class OtherElementType, class OtherExtents, class OtherLayoutPolicy>
119 template<
class... IndexType>
125 template<
class IndexType,
size_t N>
132 static constexpr
int rank() noexcept
133 {
return extents_type::rank(); }
137 {
return extents_type::static_extent(k); }
140 {
return m_map.extents(); }
142 {
return extents().extent(k); }
148 for (std::size_t k=0; k<
rank(); ++k)
162 {
return mapping_type::is_always_unique(); }
164 {
return mapping_type::is_always_contiguous(); }
166 {
return mapping_type::is_always_strided(); }
171 {
return mapping().is_unique(); }
173 {
return mapping().is_contiguous(); }
175 {
return mapping().is_strided(); }
177 {
return mapping().stride(k); }
184 template<
class T, ptrdiff_t... Extents>
189 #endif // otbMdSpan_h
constexpr reference operator()(std::array< IndexType, N > const &indices) const noexcept
constexpr basic_mdspan() noexcept=default
constexpr basic_mdspan(pointer p, narrow, IndexType... dynamic_extents) noexcept
constexpr Span< element_type > span() const noexcept
constexpr basic_mdspan(const basic_mdspan< OtherElementType, OtherExtents, OtherLayoutPolicy > &other) noexcept
constexpr index_type stride(vcl_size_t k) const noexcept
static constexpr bool is_always_unique() noexcept
constexpr basic_mdspan(pointer p, mapping_type const &m) noexcept
constexpr basic_mdspan(pointer p, std::array< IndexType, N > const &dynamic_extents) noexcept
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
constexpr pointer data() const noexcept
static constexpr int rank_dynamic() noexcept
constexpr index_type unique_size() const noexcept
constexpr extents_type extents() const noexcept
constexpr basic_mdspan & operator=(basic_mdspan const &) noexcept=default
constexpr mapping_type mapping() const noexcept
static constexpr index_type static_extent(vcl_size_t k) noexcept
constexpr bool is_strided() const noexcept
ptrdiff_t difference_type
static constexpr bool is_always_contiguous() noexcept
typename layout_type::template mapping< extents_type > mapping_type
constexpr bool is_contiguous() const noexcept
constexpr index_type size() const noexcept
constexpr index_type extent(vcl_size_t k) const noexcept
constexpr reference operator[](index_type) const noexcept
std::remove_cv_t< element_type > value_type
dynamic_extent layout_type
static constexpr int rank() noexcept
constexpr std::vcl_size_t rank_dynamic() noexcept
static constexpr bool is_always_strided() noexcept
dynamic_extent extents_type
constexpr reference operator()(IndexType... indices) const noexcept
constexpr bool is_unique() const noexcept