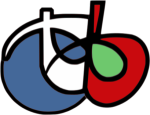 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbLineSegmentDetector_h
22 #define otbLineSegmentDetector_h
28 #include "itkUnaryFunctorImageFilter.h"
29 #include "itkGradientRecursiveGaussianImageFilter.h"
30 #include "itkGradientImageFilter.h"
42 template <
class TInputPixel,
class TOutputPixel>
43 class MagnitudeFunctor
48 return static_cast<TOutputPixel
>(2 * std::sqrt(input[0] * input[0] + input[1] * input[1]));
58 template <
class TInputPixel,
class TOutputPixel>
59 class OrientationFunctor
64 return static_cast<TOutputPixel
>(std::atan2(input[0], -input[1]));
91 template <
class TInputImage,
class TPrecision =
double>
111 typedef typename InputImageType::SizeType
SizeType;
171 using Superclass::SetInput;
179 return m_UsedPointImage;
183 return m_MagnitudeFilter->GetOutput();
187 return m_OrientationFilter->GetOutput();
197 void GenerateInputRequestedRegion()
override;
200 void GenerateData()
override;
206 virtual CoordinateHistogramType SortImageByModulusValue(MagnitudeImagePointerType modulusImage);
209 virtual void LineSegmentDetection(CoordinateHistogramType& CoordinateHistogram);
212 virtual bool IsNotUsed(InputIndexType& index)
const;
215 virtual bool IsUsed(InputIndexType& index)
const;
218 virtual bool IsNotIni(InputIndexType& index)
const;
221 virtual void SetPixelToUsed(InputIndexType index);
224 virtual void SetPixelToNotIni(InputIndexType index);
227 virtual void SetRegionToNotIni(IndexVectorType region);
230 virtual bool GrowRegion(InputIndexType index, IndexVectorType& region,
double& regionAngle);
233 virtual bool IsAligned(
double Angle,
double regionAngle,
double prec)
const;
236 virtual int ComputeRectangles();
239 virtual RectangleType Region2Rectangle(IndexVectorType region,
double regionAngle);
242 virtual double ComputeRegionOrientation(IndexVectorType region,
double x,
double y,
double angleRegion)
const;
245 virtual double angle_diff(
double a,
double b)
const;
248 virtual double ComputeRectNFA(
const RectangleType& rec)
const;
251 virtual double ImproveRectangle(RectangleType& rectangle)
const;
254 virtual double NFA(
int n,
int k,
double p,
double logNT)
const;
257 virtual void CopyRectangle(RectangleType& rDst, RectangleType& rSrc)
const;
260 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
264 void operator=(
const Self&) =
delete;
287 #ifndef OTB_MANUAL_INSTANTIATION
MagnitudeFilterPointerType m_MagnitudeFilter
GradientFilterType::OutputImageType GradientOutputImageType
VectorDataType::DataNodeType DataNodeType
DirectionVectorType m_DirectionVector
OrientationFilterType::Pointer OrientationFilterPointerType
itk::UnaryFunctorImageFilter< GradientOutputImageType, OutputImageType, Functor::OrientationFunctor< typename GradientOutputImageType::PixelType, TPrecision > > OrientationFilterType
OrientationFilterPointerType m_OrientationFilter
InputImageType::RegionType RegionType
OutputImageDirType::Pointer GetGradOri()
VectorDataType::PointType PointType
This functor computes the orientation of a cavariant vector Orientation values lies between 0 and 2*...
RectangleListType::iterator RectangleListTypeIterator
VectorDataSource< VectorData< TPrecision > > Superclass
MagnitudeImageType::Pointer MagnitudeImagePointerType
TOutputPixel operator()(const TInputPixel &input)
TInputImage InputImageType
std::vector< float > DirectionVectorType
CoordinateHistogramType::iterator CoordinateHistogramIteratorType
this class implement a fast line detector with false detection control using the a contrario method
MagnitudeFilterType::OutputImageType MagnitudeImageType
This class represents a hierarchy of vector data.
itk::SmartPointer< Self > Pointer
Superclass::IndexType IndexType
This class represents a node of data in a vector data hierarchy.
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
itk::GradientRecursiveGaussianImageFilter< OutputImageType > GradientFilterType
Creation of an "otb" image which contains metadata.
OutputImageType::IndexType OutputIndexType
MagnitudeImagePointerType GetGradMod()
otb::Image< unsigned char, 2 > LabelImageType
InputImageType::SizeType SizeType
unsigned int m_MinimumRegionSize
Image< TPrecision, 2 > OutputImageType
InputImageType::SpacingType SpacingType
std::vector< IndexVectorType > VectorOfIndexVectorType
LabelImageType::Pointer LabelImagePointerType
std::vector< RectangleType > RectangleListType
OutputImageType::SizeType OutputSizeType
InputImageType::PixelType InputPixelType
InputImageType::PointType OriginType
GradientFilterType::Pointer GradientFilterPointerType
LineType::VertexType VertexType
VectorData< TPrecision > VectorDataType
itk::UnaryFunctorImageFilter< GradientOutputImageType, OutputImageType, Functor::MagnitudeFunctor< typename GradientOutputImageType::PixelType, TPrecision > > MagnitudeFilterType
DataNodeType::PointType PointType
VectorOfIndexVectorType m_RegionList
RectangleType::iterator RectangleIteratorType
LabelImagePointerType m_UsedPointImage
GradientFilterPointerType m_GradientFilter
VectorDataType::LineType LineType
InputImageType::IndexType InputIndexType
double m_DirectionsAllowed
This class implement a PolyLineParametricPath for which a value can be set. The value is stored in th...
DirectionVectorType::iterator DirectionVectorIteratorType
TOutputPixel operator()(const TInputPixel &input)
This functor computes the magnitude of a covariant vector.
OutputImageType::PixelType OutputPixelType
~LineSegmentDetector() override
OrientationFilterType::OutputImageType OutputImageDirType
Superclass::VertexType VertexType
OutputImageDirType::RegionType OutputImageDirRegionType
itk::SmartPointer< const Self > ConstPointer
RectangleListType m_RectangleList
Superclass::PixelType PixelType
Filter hierarchy for generating VectorData.
itk::SmartPointer< Self > Pointer
MagnitudeFilterType::OutputImageType::PixelType MagnitudePixelType
std::vector< double > RectangleType
std::vector< OutputIndexType > IndexVectorType
std::vector< IndexVectorType > CoordinateHistogramType
IndexVectorType::iterator IndexVectorIteratorType
MagnitudeFilterType::Pointer MagnitudeFilterPointerType
LabelImagePointerType GetMap()
Superclass::SizeType SizeType