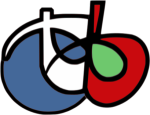 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
22 #ifndef otbImageSimulationMethod_h
23 #define otbImageSimulationMethod_h
26 #include "itkImageSource.h"
36 #include "itkResampleImageFilter.h"
38 #include "itkLabelMapToLabelImageFilter.h"
62 template <
class TInputVectorData,
class TSpatialisation,
class TSimulationStep1,
class TSimulationStep2,
class TFTM,
class TOutputImage>
82 typedef typename SpatialisationType::OutputLabelMapType
LabelMapType;
83 typedef typename LabelMapType::LabelObjectType::LabelType
LabelType;
94 typedef typename OutputImageType::IndexType
IndexType;
111 typedef itk::ResampleImageFilter<SingleImageType, SingleImageType, double>
FTMFilterType;
125 itkSetMacro(NumberOfComponentsPerPixel,
unsigned int);
127 itkGetMacro(NumberOfComponentsPerPixel,
unsigned int);
131 itkSetMacro(Radius,
unsigned int);
133 itkGetMacro(Radius,
unsigned int);
136 itkSetMacro(SatRSRFilename, std::string);
138 itkGetMacro(SatRSRFilename, std::string);
141 itkSetMacro(PathRoot, std::string);
143 itkGetMacro(PathRoot, std::string);
146 itkSetMacro(Mean,
double);
148 itkGetMacro(Mean,
double);
151 itkSetMacro(Variance,
double);
153 itkGetMacro(Variance,
double);
183 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
186 void GenerateOutputInformation()
override;
190 void operator=(
const Self&) =
delete;
215 #ifndef OTB_MANUAL_INSTANTIATION
SimulationStep2Type::Pointer SimulationStep2Pointer
itk::ImageSource< TOutputImage > Superclass
ImageSimulationMethod Self
TSimulationStep1 SimulationStep1Type
InterpolatorType::Pointer InterpolatorPointer
ImageListPointer m_ImageList
OutputImageType::IndexType IndexType
OutputImageType::InternalPixelType InternalPixelType
InputVectorDataPointer m_InputVectorData
std::vector< InterpolatorPointer > m_InterpolatorList
otb::LabelMapToSimulatedImageFilter< LabelMapType, SimulationStep1Type, SimulationStep2Type, OutputImageType > LabelMapToSimulatedImageFilterType
LabelMapToLabelImageFilterType::Pointer LabelMapToLabelImageFilterPointer
TSpatialisation SpatialisationType
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
Creation of an "otb" image which contains metadata.
SimulationStep1Type::Pointer SimulationStep1Pointer
Transform a labelMap into an image.
itk::SmartPointer< Self > Pointer
~ImageSimulationMethod() override
itk::LabelMapToLabelImageFilter< LabelMapType, OutputLabelImageType > LabelMapToLabelImageFilterType
TSimulationStep2 SimulationStep2Type
ImageListToVectorImageFilterType::Pointer ImageListToVectorImageFilterPointer
ImageListType::Pointer ImageListPointer
Converts an ImageList to a VectorImage.
LabelMapToSimulatedImageFilterType::Pointer LabelMapToSimulatedImageFilterPointer
LabelMapType::LabelObjectType::LabelType LabelType
InputVectorDataType::ConstPointer InputVectorDataConstPointer
LabelMapToSimulatedImageFilterPointer m_LabelMapToSimulatedImageFilter
std::vector< MultiToMonoChannelFilterPointer > m_MultiToMonoChannelFilterList
itk::SmartPointer< Self > Pointer
itk::ResampleImageFilter< SingleImageType, SingleImageType, double > FTMFilterType
otb::ProlateInterpolateImageFunction< SingleImageType > InterpolatorType
itk::SmartPointer< Self > Pointer
MultiToMonoChannelFilterType::Pointer MultiToMonoChannelFilterPointer
unsigned int m_NumberOfComponentsPerPixel
TInputVectorData InputVectorDataType
ImageListToVectorImageFilterPointer m_ImageListToVectorImageFilter
FTMType::Pointer FTMPointer
OutputImageType::ConstPointer OutputImageConstPointer
OutputImageType::Pointer OutputImagePointer
otb::ImageListToVectorImageFilter< ImageListType, OutputImageType > ImageListToVectorImageFilterType
itk::SmartPointer< Self > Pointer
otb::Image< LabelType, 2 > OutputLabelImageType
LabelMapToLabelImageFilterPointer m_LabelMapToLabelImageFilter
This class represent a list of images.
SpatialisationType::OutputLabelMapType LabelMapType
SpatialisationType::Pointer SpatialisationPointer
FTMFilterType::Pointer FTMFilterPointer
std::string m_SatRSRFilename
std::vector< FTMFilterPointer > m_FTMFilterList
SpatialisationPointer m_Spatialisation
InputVectorDataType::Pointer InputVectorDataPointer
itk::SmartPointer< Self > Pointer
MultiToMonoChannelFilterType::OutputImageType SingleImageType
otb::ImageList< SingleImageType > ImageListType
itk::SmartPointer< const Self > ConstPointer
TOutputImage OutputImageType
otb::MultiToMonoChannelExtractROI< double, double > MultiToMonoChannelFilterType
Prolate interpolation of an otb::image.